# Computer vision
# Workshop 1
# Opdracht 2F
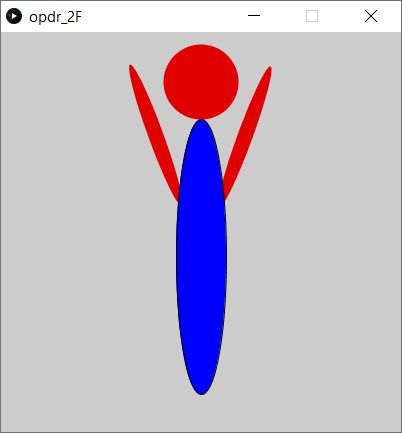
void setup () {
size(400, 400);
noStroke();
fill(225,0,0);
ellipse(200, 50, 75, 75);
rotate(0.35);
fill(225,0,0);
ellipse(265, 15, 15, 150);
rotate(-0.7);
ellipse(110, 150, 15, 150);
rotate(0.35);
fill(0,0,255);
stroke(0);
ellipse(200, 225, 50, 275);
}
# Opdracht 2I
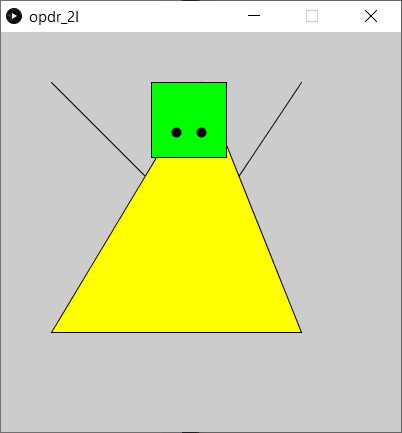
void setup () {
size(400, 400);
noStroke();
fill(225,0,0);
stroke(0);
line(50, 50, 200, 200);
line(200, 200, 300, 50);
fill(255,255,0);
triangle(300, 300, 50, 300, 200, 50);
fill(0,255,0);
rect(150, 50, 75, 75);
strokeWeight(10);
point(175, 100);
point(200, 100);
}
# Opdracht 2O
void drawPuppet () {
background(255);
noStroke();
strokeWeight(2);
fill(225, 0, 0);
stroke(0);
line(250, 200, offsetX+10, offsetY);
line(150, 200, offsetX-10, offsetY);
fill(255, 255, 0);
triangle(300, 300, 50, 300, 200, 50);
fill(0, 255, 0);
rect(150, 50, 75, 75);
strokeWeight(10);
point(160 + (offsetX / 200) *18, 80 + 20 * offsetY / 400);
point(185 + (offsetX / 200) * 18, 80 + 20 * offsetY / 400);
fill(255);
stroke(255,0,0);
ellipse(offsetX, offsetY, 50, 50);
}
void setup () {
size(400, 400);
frameRate(60);
drawPuppet();
}
float offsetX = 1;
float offsetY = 1;
void draw () {
// Check if greater than 0 otherwise there will be an error because dividing by 0 seems difficult for this program
// Computer looks dumb that way, because I can do that math easily :D
if (mouseX > 0) offsetX = mouseX;
if (mouseY > 0) offsetY = mouseY;
drawPuppet();
}
# Opdracht 3B
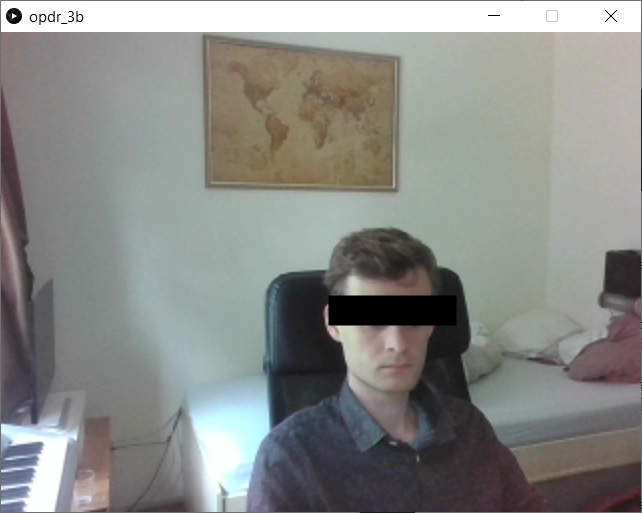
import gab.opencv.*;
import processing.video.*;
import java.awt.*;
Capture video;
OpenCV opencv;
PImage img;
void setup() {
size(640, 480);
video = new Capture(this, 640/2, 480/2);
opencv = new OpenCV(this, 640/2, 480/2);
opencv.loadCascade(OpenCV.CASCADE_FRONTALFACE);
img = loadImage("switch3730.png");
video.start();
}
void draw() {
scale(2);
opencv.loadImage(video);
image(video, 0, 0 );
fill(0,0,0);
stroke(0, 0, 0);
strokeWeight(3);
Rectangle[] faces = opencv.detect();
println(faces.length);
for (int i = 0; i < faces.length; i++) {
float w = faces[i].width;
println(faces[i].x + "," + faces[i].y);
rect(faces[i].x, faces[i].y + 12, faces[i].width, 12);
//image(img, faces[i].x, faces[i].y+faces[i].height/2.8, faces[i].width, w / 702 * 443);
}
}
void captureEvent(Capture c) {
c.read();
}
# Opdracht 3D
import gab.opencv.*;
import processing.video.*;
import java.awt.*;
Capture video;
OpenCV opencv;
PImage img;
void setup() {
size(640, 480);
video = new Capture(this, 640/2, 480/2);
opencv = new OpenCV(this, 640/2, 480/2);
opencv.loadCascade(OpenCV.CASCADE_FRONTALFACE);
img = loadImage("switch3730.png");
video.start();
}
void draw() {
scale(2);
opencv.loadImage(video);
image(video, 0, 0 );
noFill();
stroke(0, 255, 0);
strokeWeight(3);
Rectangle[] faces = opencv.detect();
println(faces.length);
for (int i = 0; i < faces.length; i++) {
float w = faces[i].width;
println(faces[i].x + "," + faces[i].y);
//rect(faces[i].x, faces[i].y, faces[i].width, faces[i].height);
image(img, faces[i].x, faces[i].y+faces[i].height/2.8, faces[i].width, w / 702 * 443);
}
}
void captureEvent(Capture c) {
c.read();
}
# Opdracht 4B
Processing
import processing.serial.*;
Serial myPort; // The serial port
void setup() {
size(200, 200);
// List all the available serial ports:
printArray(Serial.list());
// Open the port you are using at the rate you want:
myPort = new Serial(this, Serial.list()[0], 9600);
}
void draw() {
textSize(72);
while (myPort.available() > 0) {
String inBuffer = myPort.readString();
if (inBuffer != null) {
clear();
text(inBuffer, 80, 128);
}
}
}
Arduino
#include <IRremote.h>
const int RECV_PIN = 7;
IRrecv irrecv(RECV_PIN);
decode_results results;
void setup() {
Serial.begin(9600);
irrecv.enableIRIn();
irrecv.blink13(true);
}
void loop() {
if (irrecv.decode(&results)) {
if (results.value == 16753245) { Serial.println("1"); }
if (results.value == 16736925) { Serial.println("2"); }
if (results.value == 16769565) { Serial.println("3"); }
if (results.value == 16720605) { Serial.println("4"); }
if (results.value == 16712445) { Serial.println("5"); }
if (results.value == 16761405) { Serial.println("6"); }
if (results.value == 16769055) { Serial.println("7"); }
if (results.value == 16754775) { Serial.println("8"); }
if (results.value == 16748655) { Serial.println("9"); }
if (results.value == 16750695) { Serial.println("0"); }
if (results.value == 16726215) { Serial.println("OK"); }
irrecv.resume();
}
}
← Arduino Guest lecture →